
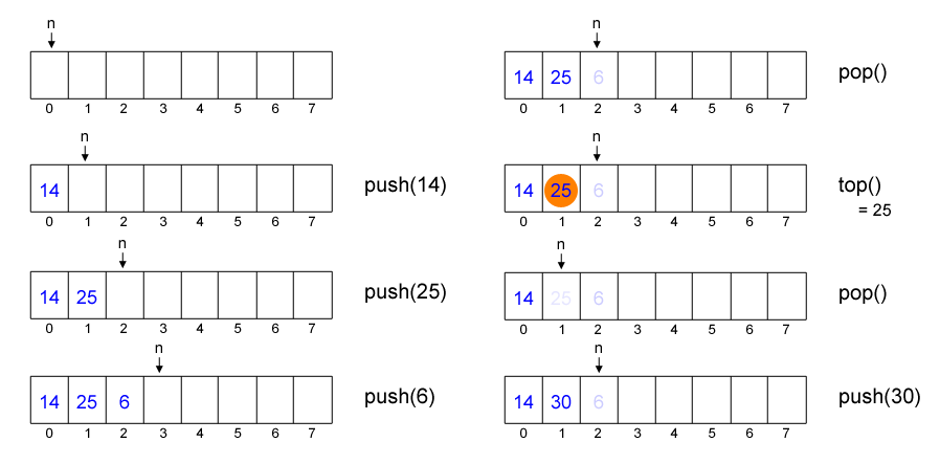
Allocate a new array B with larger capacity (A. If an element is appended to a list at a time, when the underlying array is full, we need to perform following steps. We can’t actually grow the array, its capacity is fixed. So deleting of the middle element can be done in O(1) if we just pop the element from the front of the deque. Dynamic Array Logic Implementation: The key is to provide means to grows an array A that stores the elements of a list. We will see that the middle element is always the front element of the deque. If after the pop operation, the size of the deque is less than the size of the stack, we pop an element from the top of the stack and insert it back into the front of the deque so that size of the deque is not less than the stack. The pop operation on myStack will remove an element from the back of the deque. The Stack is an abstract data type that demonstrates Last in first out ( LIFO) behavior. In this program, we will see how to implement stack using Linked List in java. The number of elements in the deque stays 1 more or equal to that in the stack, however, whenever the number of elements present in the deque exceeds the number of elements in the stack by more than 1 we pop an element from the front of the deque and push it into the stack. Implement stack using Linked List in java If you want to practice data structure and algorithm programs, you can go through 100+ java coding interview questions. Insert operation on myStack will add an element into the back of the deque.
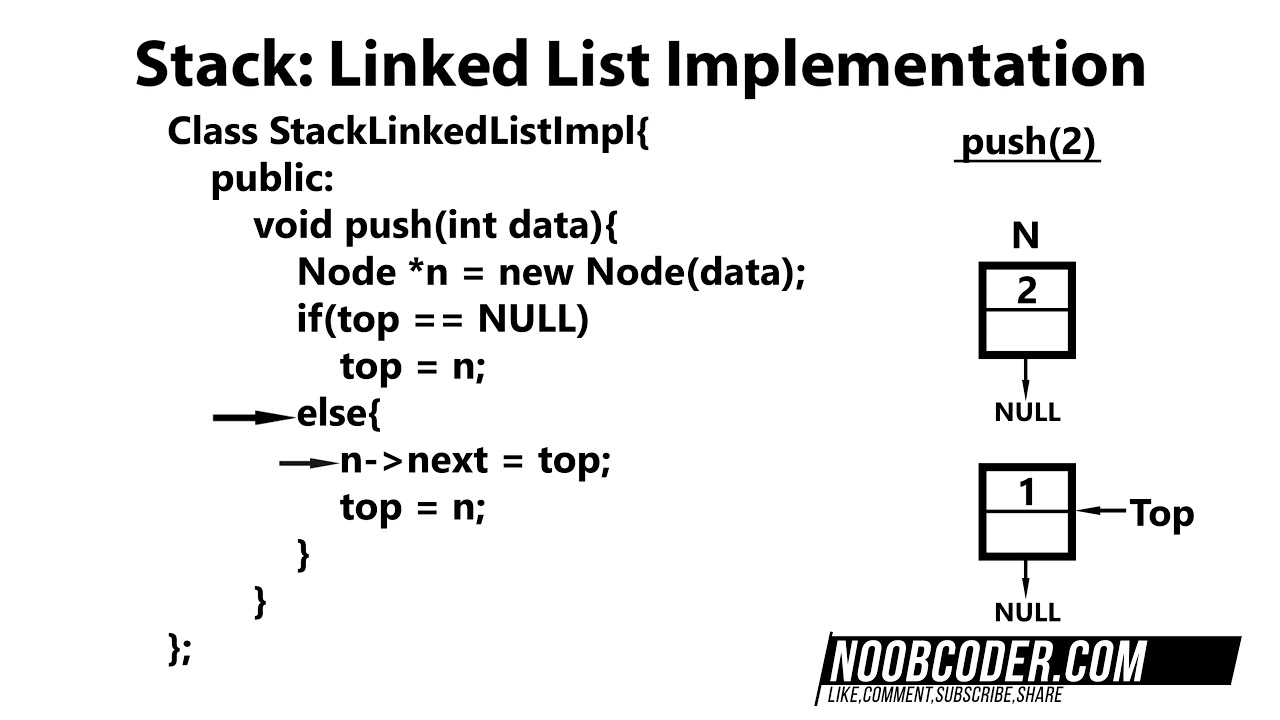
We will use a standard stack to store half of the elements and the other half of the elements which were added recently will be present in the deque. Evaluate run-time overhead incurred using recursive versus iterative processes. Method 2: Using a standard stack and a deque This method makes sure that oldest entered element is always at the top of stack 1, so that deQueue operation just pops from stack1. Explain and implement recursive algorithms and demonstrate how the run-time stack makes this possible. ISRO CS Syllabus for Scientist/Engineer Exam.ISRO CS Original Papers and Official Keys.GATE CS Original Papers and Official Keys.Queues are better suited for linked lists, but it’s a fairly common interview question to implement a queue using a stack. The pop and push methods are built in and have a time complexity of O(1). This is why when implementing a stack in JavaScript, we can simply use an array. This is often described by using the acronyms FIFO (First In, First Out) and LIFO (Last In, Last Out). To insert into the middle somewhere, pop items from the 'list' stack and push them onto the. To remove from the head, pop from the stack. To add an item at the head, simply push the item onto the stack. One stack is the 'list,' and the other is used for temporary storage. With stacks, we add items to the end and remove them from the end, but with queues we add to the end but remove from the beginning. Some common data structures include arrays, linked lists, hash tables, heaps, trees, tries, stacks. You can simulate a linked list by using two stacks. They really aren’t all that different, both are data structures in which we only append elements, which is to say, we add them to the end. Queue Implementation Techniques As with the stack, the two most common implementation techniques for a queue are to use a linked list or to use an array. For a more complete list of the bug fixes included in this release. linked lists, stacks and queues), and the advantages and disadvantages of the. The TLSv1.3 implementation is available in JDK 8u from 8u261 and enabled by default.
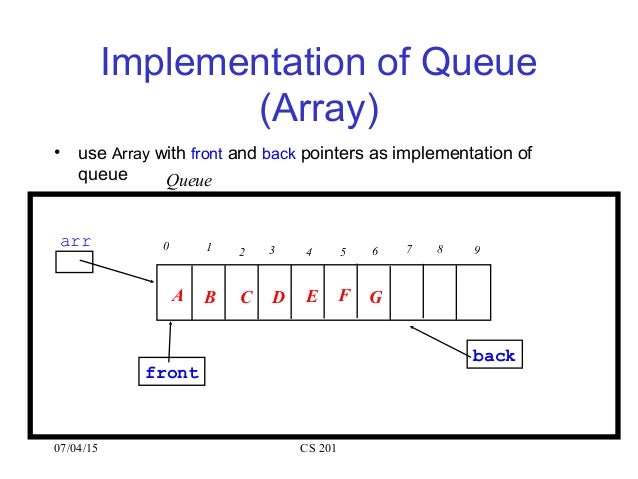
We should start with the difference between a stack and a queue. The task of implementing the discussed algorithms as computer programs is. Full disclosure: I was asked to do this in an interview, and am clearing some things up for myself and hopefully you as well. Continuing my series of writing blogs about things in JavaScript that are tricky to me initially, this is a blog explaining a simple way of building a stack using queues in JavaScript.
